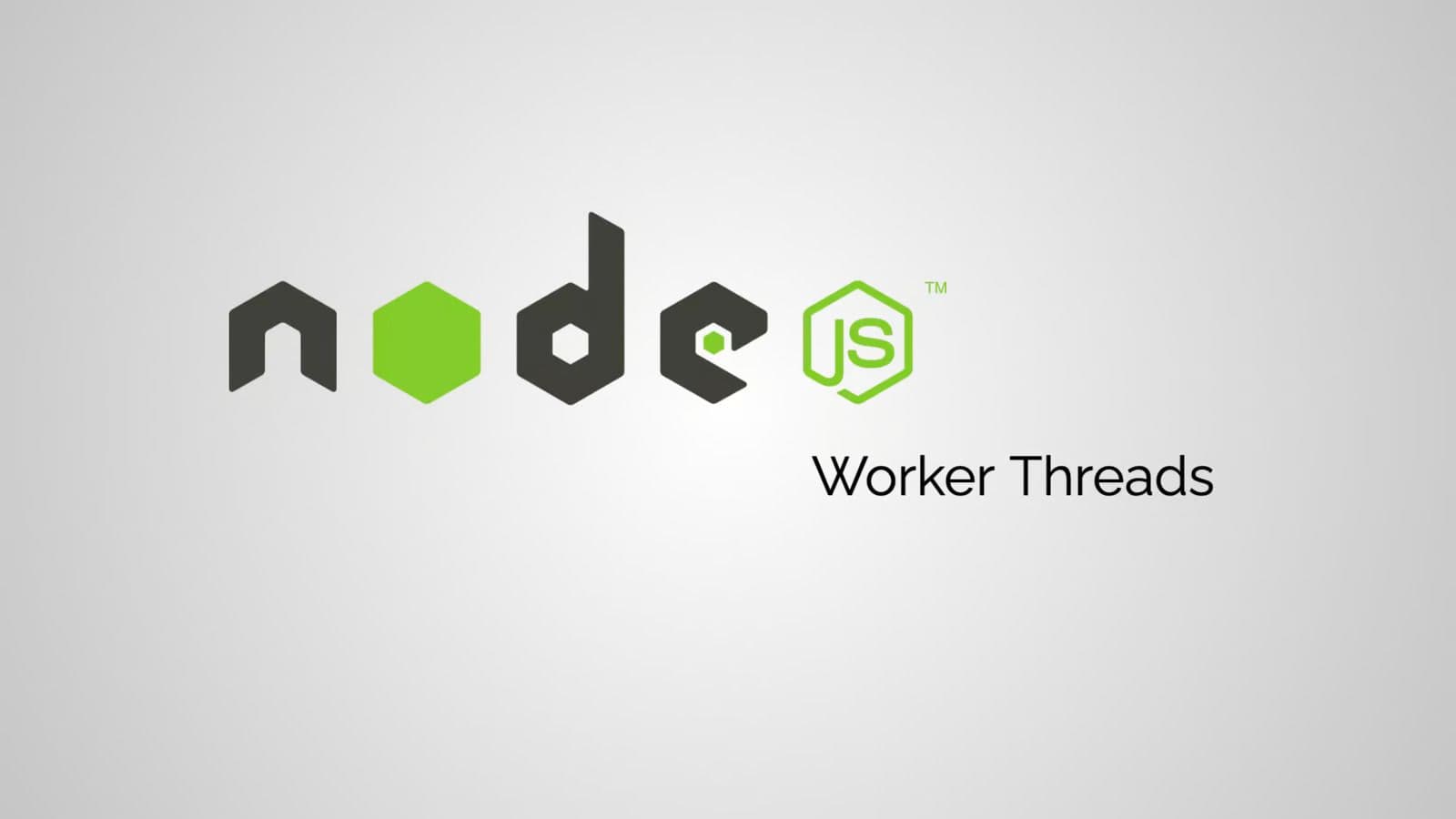
Node.js is a powerful platform for building scalable applications, but sometimes, certain operations can be computationally intensive and block the event loop, impacting performance. Image processing is one such task that can benefit from parallel execution. In this article, we'll explore how to leverage Node.js' worker_threads module to process images asynchronously, improving performance and responsiveness.
Understanding Worker Threads
Node.js provides a module called worker_threads that allows you to run JavaScript code in separate threads. This enables you to perform CPU-intensive tasks in parallel, freeing up the main event loop for other operations.
Setting Up the Project
First, let's set up a basic Node.js project. Ensure you have Node.js installed on your machine. Create a new directory for your project and run npm init to initialize a new Node.js project. Install the sharp package, which we'll use for image processing:
mkdir image-processing-app
cd image-processing-app
npm init -y
npm install sharp
Integrating with an HTTP Server
To integrate the image processing functionality into an HTTP server, we'll create an endpoint that accepts image processing requests and returns the processed image:
//server.js
const http = require('http');
const fs = require('fs');
const { Worker } = require('worker_threads');
const server = http.createServer((req, res) => {
if (req.url === '/process-image' && req.method === 'POST') {
let body = '';
req.on('data', (chunk) => {
body += chunk;
});
req.on('end', async () => {
try {
const imageData = JSON.parse(body);
const result = await processImageWithWorker(imageData);
res.writeHead(200, { 'Content-Type': 'application/json' });
res.end(JSON.stringify({ result }));
} catch (error) {
res.writeHead(500, { 'Content-Type': 'application/json' });
res.end(JSON.stringify({ error: 'Internal Server Error' }));
}
});
} else {
res.writeHead(404, { 'Content-Type': 'application/json' });
res.end(JSON.stringify({ error: 'Not Found' }));
}
});
server.listen(3000, () => {
console.log('Server running on port 3000');
});
function processImageWithWorker(imageData) {
return new Promise((resolve, reject) => {
const worker = new Worker('./worker.js', { workerData: imageData });
worker.on('message', (result) => {
const buffer = Buffer.from(result, 'base64');
const fileName = `output_${Date.now()}.jpg`;
fs.writeFileSync(fileName, buffer);
console.log(`Processed image saved to ${fileName}`);
resolve(fileName);
});
worker.on('error', (error) => {
reject(error);
});
});
}
Using Worker Threads for Image Processing
Now, let's use the worker_threads module to create a worker thread for image processing. This will allow us to offload image processing tasks to the worker thread, keeping the main thread free for handling other requests:
//worker.js
const { parentPort, workerData } = require('worker_threads');
const sharp = require('sharp');
const processImage = async (imageData) => {
const { imagePath, width, height } = imageData;
try {
const resizedImage = await sharp(imagePath).resize(width, height).toBuffer();
return resizedImage.toString('base64');
} catch (error) {
console.error('Error processing image:', error);
return null;
}
};
processImage(workerData).then((result) => {
parentPort.postMessage(result);
}).catch((error) => {
console.error('Error in Worker Thread:', error);
});
Testing
To test the image processing functionality using Postman, follow these steps:
1.Start the Server: Make sure your Node.js server is running. If not, start it using the command node server.js (assuming your server file is named server.js).
2.Prepare the Request:
- Set the request method to POST.
- Set the request URL to http://localhost:3000/process-image.
- Set the request body to JSON format with the following structure:
{
"imagePath": "path/to/your/image.jpg",
"width": 100,
"height": 100
}
3.Send the Request: Click the "Send" button to send the request to your server.
4.Check the Response: You should receive a response from the server containing the processed image data. If there are any errors, the server will respond with an error message.

5.Verify the Processed Image: You can verify that the image was processed correctly by opening the file saved by the server (the file name will be printed in the console).

Conclusion
By using Node.js with worker threads, we can improve the performance of our applications by offloading CPU-intensive tasks like image processing to separate threads. This allows the main event loop to remain responsive, leading to a better user experience. Experiment with this approach in your Node.js projects to see the performance benefits for yourself!
Happy Coding! 🚀