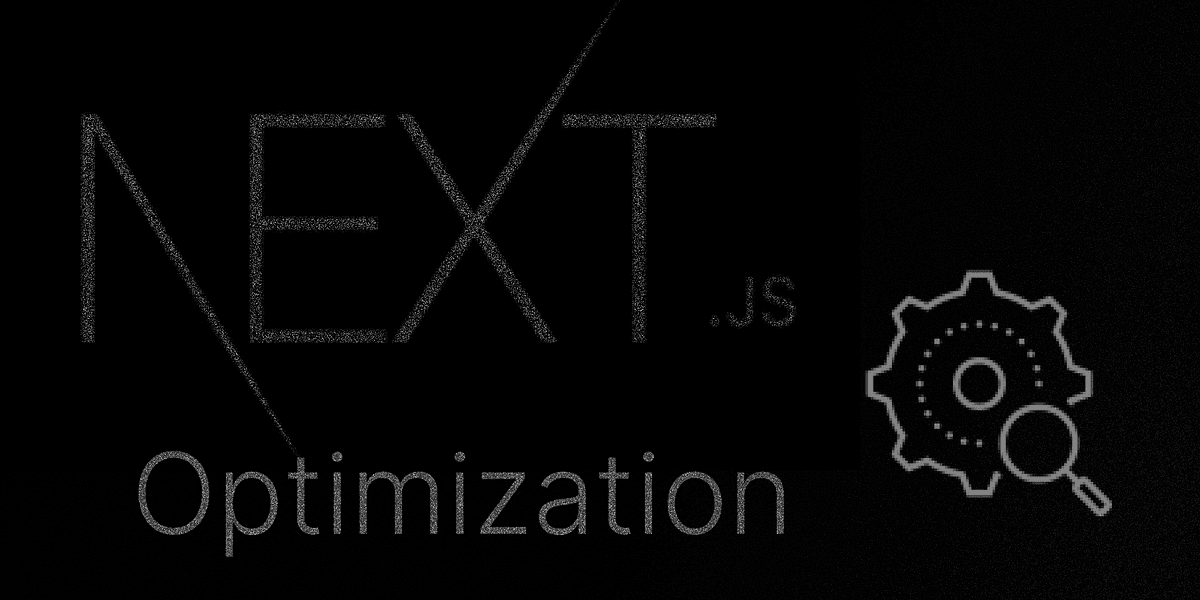
Understanding Lighthouse Scores
Before we dive into optimization techniques, let's understand Lighthouse scores. Lighthouse is an open-source tool from Google that audits web pages for performance, accessibility, SEO, and more. A Lighthouse score ranges from 0 to 100, with higher scores indicating better performance.
1.Use Static Site Generation (SSG)
Next.js excels in server-side rendering, but not all content needs SSR. For static content, use Static Site Generation (SSG) to generate HTML at build time, reducing server load and improving performance.
export async function getStaticProps() {
// Fetch data from API or filesystem
const data = await fetchData();
return {
props: {
data,
},
revalidate: 60, // Regenerate page every 60 seconds
};
}
2. Leverage Code Splitting and Lazy Loading
Break your application into smaller chunks and only load what's necessary for the initial render. Next.js supports code splitting, allowing you to dynamically import components or pages as needed.
// Import the dynamic function from Next.js
import dynamic from 'next/dynamic';
// Define a new component called DynamicComponent
const DynamicComponent = dynamic(() => import('../components/DynamicComponent'), {
// Specify a loading component to show while the dynamic component is loading
loading: () => <p>Loading...</p>,
//Disable SSR for the component
ssr:false
});
3.Optimize Images
Large image files can slow down your website. Next.js provides an Image component that optimizes images based on device size and resolution.
// Import the Image component from Next.js
import Image from 'next/image';
function MyComponent() {
return (
<Image
// Specify the image source
src="/image.jpg"
// Provide a descriptive alt text for accessibility
alt="Description of image"
// Set the width of the image
width={500}
// Set the height of the image
height={300}
/>
);
}
4. Utilize Content Delivery Networks (CDNs) and Caching
CDNs distribute assets globally, reducing latency. Implement caching strategies to minimize server requests and improve load times for returning visitors.
// Example with caching using SWR library
import useSWR from 'swr';
function MyComponent() {
const { data, error } = useSWR('/api/data', fetcher, {
refreshInterval: 30000,
});
if (error) return <div>Error fetching data</div>;
if (!data) return <div>Loading...</div>;
return <div>{data}</div>;
}
Conclusion
By implementing these advanced Next.js performance optimization techniques, you can significantly improve your website's speed and achieve a perfect 100 Lighthouse score. Continuously monitor and fine-tune your optimizations to deliver exceptional web experiences. With Next.js, blazing-fast websites are within your grasp.
Happy optimizing! 🚀